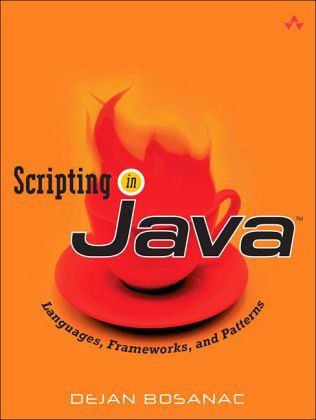
Scripting in Java
Languages, Frameworks, and Patterns
Versandkostenfrei!
Nicht lieferbar
Scripting languages have played an important role in the information technology infrastructure for many years. They have been used for all kinds of tasks, ranging from job automation to prototyping and implementation of complex software projects. The Java development platform can also benefit from scripting concepts and languages. Java developers can use scripting languages in areas that are proven to be the most suitable for this technology. This synergy of the Java platform and scripting languages adds an extra quality to the overall software development process. As more Java developers are coming from web-oriented (i.e., HTML, JavaScript, a bit of PHP or JSP) backgrounds, more Java developers are turning to scripting as an option to increase code efficiency within their Java applications. In this book Bosanac describes the concepts behind scripting languages, summarizes solutions available to Java developers, and explores use cases and design patterns for applying scripting languages in Java applications. Product Description
Groovy and Beyond: Leverage the Full Power of Scripting on the JavaTM Platform!
Using the JavaTM platform's new scripting support, you can improve efficiency, streamline your development processes, and solve problems ranging from prototyping to Web application programming. In Scripting in Java, Dejan Bosanac covers key aspects of scripting with Java, from the exciting new Groovy scripting language to Java's new Scripting and Web Scripting APIs.
Bosanac begins by reviewing the role and value of scripting languages, and then systematically introduces today's best scripting solutions for the Java platform. He introduces Java scripting frameworks, identifies proven patterns for integrating scripting into Java applications, and presents practical techniques for everything from unit testing to project builds. He supports key concepts with extensive code examples that demonstrate scripting at work in real-world Java projects. Coverage includes
· Why scripting languages offer surprising value to Java programmers
· Scripting languages that run inside the JVM: BeanShell, JavaScript, and Python
· Groovy in depth: installation, configuration, Java-like syntax, Java integration, security, and more
· Groovy extensions: accessing databases, working with XML, and building simple Web applications and Swing-based UIs
· Bean Scripting Framework: implementation, basic abstractions, and usage examples
· Traditional and new patterns for Java-based scripting
· JSR 223 Scripting API: language bindings, discovery mechanisms, threading, pluggable namespaces, and more
· JSR 223 Web Scripting Framework: scripting the generation of Web content within servlet containers
About the Web Site
All code examples are available for download at this book's companion Web site.
Backcover
Groovy and Beyond: Leverage the Full Power of Scripting on the JavaTM Platform!
Using the JavaTM platform's new scripting support, you can improve efficiency, streamline your development processes, and solve problems ranging from prototyping to Web application programming. In Scripting in Java, Dejan Bosanac covers key aspects of scripting with Java, from the exciting new Groovy scripting language to Java's new Scripting and Web Scripting APIs.
Bosanac begins by reviewing the role and value of scripting languages, and then systematically introduces today's best scripting solutions for the Java platform. He introduces Java scripting frameworks, identifies proven patterns for integrating scripting into Java applications, and presents practical techniques for everything from unit testing to project builds. He supports key concepts with extensive code examples that demonstrate scripting at work in real-world Java projects. Coverage includes
· Why scripting languages offer surprising value to Java programmers
· Scripting languages that run inside the JVM: BeanShell, JavaScript, and Python
· Groovy in depth: installation, configuration, Java-like syntax, Java integration, security, and more
· Groovy extensions: accessing databases, working with XML, and building simple Web applications and Swing-based UIs
· Bean Scripting Framework: implementation, basic abstractions, and usage examples
· Traditional and new patterns for Java-based scripting
· JSR 223 Scripting API: language bindings, discovery mechanisms, threading, pluggable namespaces, and more
· JSR 223 Web Scripting Framework: scripting the generation of Web content within servlet containers
About the Web Site
All code examples are available for download at this book's companion Web site.
PREFACE XVII
PART I 1
CHAPTER 1 INTRODUCTION TO SCRIPTING 3
BACKGROUND 4
DEFINITION OF A SCRIPTING LANGUAGE 8
COMPILERS VERSUS INTERPRETERS 8
SOURCE CODE IN PRODUCTION 12
TYPING STRATEGIES 13
DATA STRUCTURES 17
CODE AS DATA 19
SUMMARY 23
SCRIPTING LANGUAGES AND VIRTUAL MACHINES 24
A COMPARISON OF SCRIPTING AND SYSTEM PROGRAMMING 26
RUNTIME PERFORMANCE 26
DEVELOPMENT SPEED 28
ROBUSTNESS 29
MAINTENANCE 32
EXTREME PROGRAMMING 33
THE HYBRID APPROACH 35
A CASE FOR SCRIPTING 37
CONCLUSION 38
CHAPTER 2 APPROPRIATE APPLICATIONS FOR SCRIPTING LANGUAGES 39
WIRING 40
UNIX SHELL LANGUAGES 41
PERL 43
TCL 43
PROTOTYPING 44
PYTHON 47
CUSTOMIZATION 49
VISUAL BASIC FOR APPLICATIONS (VBA) 50
SOFTWARE DEVELOPMENT SUPPORT 51
PROJECT BUILDING 51
TESTING 53
ADMINISTRATION AND MANAGEMENT 55
USER INTERFACE PROGRAMMING 58
TK 58
USE CASES 59
WEB APPLICATIONS 59
SCRIPTING AND UNIX 68
SCRIPTING IN GAMES 68
ADDITIONAL CHARACTERISTICS 69
EMBEDDABLE 70
EXTENSIBLE 70
EASY TO LEARN AND USE 71
CONCLUSION 72
PART II 75
CHAPTER 3 SCRIPTING LANGUAGES INSIDE THE JVM 77
UNDER THE HOOD 80
SCRIPTING LANGUAGE CONCEPTS 82
BEANSHELL 83
GETTING STARTED 83
BASIC SYNTAX 86
LOOSELY TYPED SYNTAX 87
SYNTAX FLAVORS 88
COMMANDS 91
METHODS 91
OBJECTS 92
IMPLEMENTING INTERFACES 93
EMBEDDING WITH JAVA 94
JYTHON 98
GETTING STARTED 98
BASIC SYNTAX 101
WORKING WITH JAVA 103
IMPLEMENTING INTERFACES 105
EXCEPTION HANDLING 107
EMBEDDING WITH JAVA 108
CONCLUSION 109
RHINO 110
GETTING STARTED 110
WORKING WITH JAVA 111
IMPLEMENTING INTERFACES 112
JAVAADAPTER 114
EMBEDDING WITH JAVA 114
HOST OBJECTS 117
CONCLUSION 120
GROOVY 120
OTHER SCRIPTING LANGUAGES 122
JRUBY 122
TCL/JAVA 122
JUDOSCRIPT 122
OBJECTSCRIPT 123
CONCLUSION 123
CHAPTER 4 GROOVY 125
WHY GROOVY? 126
INSTALLATION 127
RUNNING GROOVY SCRIPTS 127
USING THE INTERACTIVE SHELL 127
USING THE INTERACTIVE CONSOLE 128
EVALUATING THE SCRIPT FILE 129
COMPILING GROOVY SCRIPTS 130
DEPENDENCIES 131
CLASSPATH 131
ANT TASK 132
SCRIPT STRUCTURE 133
COMMAND-LINE ARGUMENTS 136
LANGUAGE SYNTAX 137
JAVA COMPATIBILITY 137
STATEMENTS 138
LOOSE TYPING 138
TYPE JUGGLING 140
STRINGS 143
GSTRINGS 145
REGULAR EXPRESSIONS 146
COLLECTIONS 148
LOGICAL BRANCHING 154
LOOPING 156
CLASSES 159
OPERATOR OVERLOADING 162
GROOVYBEANS 165
CLOSURES 168
SYSTEM OPERATIONS 178
FILES 178
PROCESSES 182
EMBEDDING WITH JAVA 184
SECURITY 190
CONCLUSION 194
CHAPTER 5 ADVANCED GROOVY PROGRAMMING 195
GROOVYSQL 196
groovy.sql.Sql 198
groovy.sql.DataSet 209
GROOVLETS 212
GROOVY TEMPLATES 220
GROOVYMARKUP 223
groovy.xml.MarkupBuilder 224
groovy.util.NodeBuilder 227
groovy.xml.SaxBuilder 230
groovy.xml.DomBuilder 232
groovy.xml.Namespace 234
groovy.util.BuilderSupport 235
GROOVY AND SWING 236
TableLayout 239
TableModel 241
CONCLUSION 243
Groovy and Beyond: Leverage the Full Power of Scripting on the JavaTM Platform!
Using the JavaTM platform's new scripting support, you can improve efficiency, streamline your development processes, and solve problems ranging from prototyping to Web application programming. In Scripting in Java, Dejan Bosanac covers key aspects of scripting with Java, from the exciting new Groovy scripting language to Java's new Scripting and Web Scripting APIs.
Bosanac begins by reviewing the role and value of scripting languages, and then systematically introduces today's best scripting solutions for the Java platform. He introduces Java scripting frameworks, identifies proven patterns for integrating scripting into Java applications, and presents practical techniques for everything from unit testing to project builds. He supports key concepts with extensive code examples that demonstrate scripting at work in real-world Java projects. Coverage includes
· Why scripting languages offer surprising value to Java programmers
· Scripting languages that run inside the JVM: BeanShell, JavaScript, and Python
· Groovy in depth: installation, configuration, Java-like syntax, Java integration, security, and more
· Groovy extensions: accessing databases, working with XML, and building simple Web applications and Swing-based UIs
· Bean Scripting Framework: implementation, basic abstractions, and usage examples
· Traditional and new patterns for Java-based scripting
· JSR 223 Scripting API: language bindings, discovery mechanisms, threading, pluggable namespaces, and more
· JSR 223 Web Scripting Framework: scripting the generation of Web content within servlet containers
About the Web Site
All code examples are available for download at this book's companion Web site.
Backcover
Groovy and Beyond: Leverage the Full Power of Scripting on the JavaTM Platform!
Using the JavaTM platform's new scripting support, you can improve efficiency, streamline your development processes, and solve problems ranging from prototyping to Web application programming. In Scripting in Java, Dejan Bosanac covers key aspects of scripting with Java, from the exciting new Groovy scripting language to Java's new Scripting and Web Scripting APIs.
Bosanac begins by reviewing the role and value of scripting languages, and then systematically introduces today's best scripting solutions for the Java platform. He introduces Java scripting frameworks, identifies proven patterns for integrating scripting into Java applications, and presents practical techniques for everything from unit testing to project builds. He supports key concepts with extensive code examples that demonstrate scripting at work in real-world Java projects. Coverage includes
· Why scripting languages offer surprising value to Java programmers
· Scripting languages that run inside the JVM: BeanShell, JavaScript, and Python
· Groovy in depth: installation, configuration, Java-like syntax, Java integration, security, and more
· Groovy extensions: accessing databases, working with XML, and building simple Web applications and Swing-based UIs
· Bean Scripting Framework: implementation, basic abstractions, and usage examples
· Traditional and new patterns for Java-based scripting
· JSR 223 Scripting API: language bindings, discovery mechanisms, threading, pluggable namespaces, and more
· JSR 223 Web Scripting Framework: scripting the generation of Web content within servlet containers
About the Web Site
All code examples are available for download at this book's companion Web site.
PREFACE XVII
PART I 1
CHAPTER 1 INTRODUCTION TO SCRIPTING 3
BACKGROUND 4
DEFINITION OF A SCRIPTING LANGUAGE 8
COMPILERS VERSUS INTERPRETERS 8
SOURCE CODE IN PRODUCTION 12
TYPING STRATEGIES 13
DATA STRUCTURES 17
CODE AS DATA 19
SUMMARY 23
SCRIPTING LANGUAGES AND VIRTUAL MACHINES 24
A COMPARISON OF SCRIPTING AND SYSTEM PROGRAMMING 26
RUNTIME PERFORMANCE 26
DEVELOPMENT SPEED 28
ROBUSTNESS 29
MAINTENANCE 32
EXTREME PROGRAMMING 33
THE HYBRID APPROACH 35
A CASE FOR SCRIPTING 37
CONCLUSION 38
CHAPTER 2 APPROPRIATE APPLICATIONS FOR SCRIPTING LANGUAGES 39
WIRING 40
UNIX SHELL LANGUAGES 41
PERL 43
TCL 43
PROTOTYPING 44
PYTHON 47
CUSTOMIZATION 49
VISUAL BASIC FOR APPLICATIONS (VBA) 50
SOFTWARE DEVELOPMENT SUPPORT 51
PROJECT BUILDING 51
TESTING 53
ADMINISTRATION AND MANAGEMENT 55
USER INTERFACE PROGRAMMING 58
TK 58
USE CASES 59
WEB APPLICATIONS 59
SCRIPTING AND UNIX 68
SCRIPTING IN GAMES 68
ADDITIONAL CHARACTERISTICS 69
EMBEDDABLE 70
EXTENSIBLE 70
EASY TO LEARN AND USE 71
CONCLUSION 72
PART II 75
CHAPTER 3 SCRIPTING LANGUAGES INSIDE THE JVM 77
UNDER THE HOOD 80
SCRIPTING LANGUAGE CONCEPTS 82
BEANSHELL 83
GETTING STARTED 83
BASIC SYNTAX 86
LOOSELY TYPED SYNTAX 87
SYNTAX FLAVORS 88
COMMANDS 91
METHODS 91
OBJECTS 92
IMPLEMENTING INTERFACES 93
EMBEDDING WITH JAVA 94
JYTHON 98
GETTING STARTED 98
BASIC SYNTAX 101
WORKING WITH JAVA 103
IMPLEMENTING INTERFACES 105
EXCEPTION HANDLING 107
EMBEDDING WITH JAVA 108
CONCLUSION 109
RHINO 110
GETTING STARTED 110
WORKING WITH JAVA 111
IMPLEMENTING INTERFACES 112
JAVAADAPTER 114
EMBEDDING WITH JAVA 114
HOST OBJECTS 117
CONCLUSION 120
GROOVY 120
OTHER SCRIPTING LANGUAGES 122
JRUBY 122
TCL/JAVA 122
JUDOSCRIPT 122
OBJECTSCRIPT 123
CONCLUSION 123
CHAPTER 4 GROOVY 125
WHY GROOVY? 126
INSTALLATION 127
RUNNING GROOVY SCRIPTS 127
USING THE INTERACTIVE SHELL 127
USING THE INTERACTIVE CONSOLE 128
EVALUATING THE SCRIPT FILE 129
COMPILING GROOVY SCRIPTS 130
DEPENDENCIES 131
CLASSPATH 131
ANT TASK 132
SCRIPT STRUCTURE 133
COMMAND-LINE ARGUMENTS 136
LANGUAGE SYNTAX 137
JAVA COMPATIBILITY 137
STATEMENTS 138
LOOSE TYPING 138
TYPE JUGGLING 140
STRINGS 143
GSTRINGS 145
REGULAR EXPRESSIONS 146
COLLECTIONS 148
LOGICAL BRANCHING 154
LOOPING 156
CLASSES 159
OPERATOR OVERLOADING 162
GROOVYBEANS 165
CLOSURES 168
SYSTEM OPERATIONS 178
FILES 178
PROCESSES 182
EMBEDDING WITH JAVA 184
SECURITY 190
CONCLUSION 194
CHAPTER 5 ADVANCED GROOVY PROGRAMMING 195
GROOVYSQL 196
groovy.sql.Sql 198
groovy.sql.DataSet 209
GROOVLETS 212
GROOVY TEMPLATES 220
GROOVYMARKUP 223
groovy.xml.MarkupBuilder 224
groovy.util.NodeBuilder 227
groovy.xml.SaxBuilder 230
groovy.xml.DomBuilder 232
groovy.xml.Namespace 234
groovy.util.BuilderSupport 235
GROOVY AND SWING 236
TableLayout 239
TableModel 241
CONCLUSION 243
Groovy and Beyond: Leverage the Full Power of Scripting on the JavaTM Platform!
Using the JavaTM platform's new scripting support, you can improve efficiency, streamline your development processes, and solve problems ranging from prototyping to Web application programming. In Scripting in Java , Dejan Bosanac covers key aspects of scripting with Java, from the exciting new Groovy scripting language to Java's new Scripting and Web Scripting APIs.
Bosanac begins by reviewing the role and value of scripting languages, and then systematically introduces today's best scripting solutions for the Java platform. He introduces Java scripting frameworks, identifies proven patterns for integrating scripting into Java applications, and presents practical techniques for everything from unit testing to project builds. He supports key concepts with extensive code examples that demonstrate scripting at work in real-world Java projects. Coverage includes
· Why scripting languages offer surprising value to Java programmers
· Scripting languages that run inside the JVM: BeanShell, JavaScript, and Python
· Groovy in depth: installation, configuration, Java-like syntax, Java integration, security, and more
· Groovy extensions: accessing databases, working with XML, and building simple Web applications and Swing-based UIs
· Bean Scripting Framework: implementation, basic abstractions, and usage examples
· Traditional and new patterns for Java-based scripting
· JSR 223 Scripting API: language bindings, discovery mechanisms, threading, pluggable namespaces, and more
· JSR 223 Web Scripting Framework: scripting the generation of Web content within servlet containers
About the Web Site
All code examples are available for download at this book's companion Web site.
Using the JavaTM platform's new scripting support, you can improve efficiency, streamline your development processes, and solve problems ranging from prototyping to Web application programming. In Scripting in Java , Dejan Bosanac covers key aspects of scripting with Java, from the exciting new Groovy scripting language to Java's new Scripting and Web Scripting APIs.
Bosanac begins by reviewing the role and value of scripting languages, and then systematically introduces today's best scripting solutions for the Java platform. He introduces Java scripting frameworks, identifies proven patterns for integrating scripting into Java applications, and presents practical techniques for everything from unit testing to project builds. He supports key concepts with extensive code examples that demonstrate scripting at work in real-world Java projects. Coverage includes
· Why scripting languages offer surprising value to Java programmers
· Scripting languages that run inside the JVM: BeanShell, JavaScript, and Python
· Groovy in depth: installation, configuration, Java-like syntax, Java integration, security, and more
· Groovy extensions: accessing databases, working with XML, and building simple Web applications and Swing-based UIs
· Bean Scripting Framework: implementation, basic abstractions, and usage examples
· Traditional and new patterns for Java-based scripting
· JSR 223 Scripting API: language bindings, discovery mechanisms, threading, pluggable namespaces, and more
· JSR 223 Web Scripting Framework: scripting the generation of Web content within servlet containers
About the Web Site
All code examples are available for download at this book's companion Web site.