David R. Brooks
C Programming: The Essentials for Engineers and Scientists (eBook, PDF)
53,95 €
53,95 €
inkl. MwSt.
Sofort per Download lieferbar
27 °P sammeln
53,95 €
Als Download kaufen
53,95 €
inkl. MwSt.
Sofort per Download lieferbar
27 °P sammeln
Jetzt verschenken
Alle Infos zum eBook verschenken
53,95 €
inkl. MwSt.
Sofort per Download lieferbar
Alle Infos zum eBook verschenken
27 °P sammeln
David R. Brooks
C Programming: The Essentials for Engineers and Scientists (eBook, PDF)
- Format: PDF
- Merkliste
- Auf die Merkliste
- Bewerten Bewerten
- Teilen
- Produkt teilen
- Produkterinnerung
- Produkterinnerung
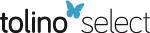
Bitte loggen Sie sich zunächst in Ihr Kundenkonto ein oder registrieren Sie sich bei
bücher.de, um das eBook-Abo tolino select nutzen zu können.
Hier können Sie sich einloggen
Hier können Sie sich einloggen
Sie sind bereits eingeloggt. Klicken Sie auf 2. tolino select Abo, um fortzufahren.
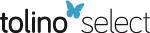
Bitte loggen Sie sich zunächst in Ihr Kundenkonto ein oder registrieren Sie sich bei bücher.de, um das eBook-Abo tolino select nutzen zu können.
This text teaches the essentials of C programming, concentrating on what readers need to know in order to produce stand-alone programs and so solve typical scientific and engineering problems. It is a learning-by-doing book, with many examples and exercises, and lays a foundation of scientific programming concepts and techniques that will prove valuable for those who might eventually move on to another language. Written for undergraduates who are familiar with computers and typical applications but are new to programming.
- Geräte: PC
- ohne Kopierschutz
- eBook Hilfe
- Größe: 66.52MB
Andere Kunden interessierten sich auch für
- Daoqi YangC++ and Object-Oriented Numeric Computing for Scientists and Engineers (eBook, PDF)53,95 €
- M. J. C. GordonThe Denotational Description of Programming Languages (eBook, PDF)40,95 €
- William F. ClocksinProgramming in Prolog (eBook, PDF)57,95 €
- David R. BrooksAn Introduction to PHP for Scientists and Engineers (eBook, PDF)36,95 €
- Generic Programming (eBook, PDF)73,95 €
- Programming Methodology (eBook, PDF)73,95 €
- Thomas W. RepsThe Synthesizer Generator Reference Manual (eBook, PDF)73,95 €
-
-
-
This text teaches the essentials of C programming, concentrating on what readers need to know in order to produce stand-alone programs and so solve typical scientific and engineering problems. It is a learning-by-doing book, with many examples and exercises, and lays a foundation of scientific programming concepts and techniques that will prove valuable for those who might eventually move on to another language. Written for undergraduates who are familiar with computers and typical applications but are new to programming.
Dieser Download kann aus rechtlichen Gründen nur mit Rechnungsadresse in A, B, BG, CY, CZ, D, DK, EW, E, FIN, F, GR, HR, H, IRL, I, LT, L, LR, M, NL, PL, P, R, S, SLO, SK ausgeliefert werden.
Produktdetails
- Produktdetails
- Verlag: Springer US
- Seitenzahl: 479
- Erscheinungstermin: 6. Dezember 2012
- Englisch
- ISBN-13: 9781461214847
- Artikelnr.: 44175884
- Verlag: Springer US
- Seitenzahl: 479
- Erscheinungstermin: 6. Dezember 2012
- Englisch
- ISBN-13: 9781461214847
- Artikelnr.: 44175884
- Herstellerkennzeichnung Die Herstellerinformationen sind derzeit nicht verfügbar.
1 Programming Preliminaries.- 1.1 A Five-Step Problem-Solving Process.- 1.2 Defining a Pseudocode Language for Algorithm Development.- 1.3 Organizing Pseudocode Into a Program.- 1.4 Examples.- 1.5 What Is the Point of Programming?.- 1.6 Your First C Program.- 2 The Basics of C Programming.- 2.1 C Program Layout.- 2.2 Basic Input and Output.- 2.3 Reading External Text Files of Unknown Length.- 2.4 Reading a File One Character at a Time.- 2.5 Applications.- 2.6 Debugging Your Programs.- 3 Data Types, Operators, and Functions.- 3.1 Specifying and Using Data Types.- 3.2 Operators.- 3.3 Type Casting.- 3.4 Intrinsic Functions.- 3.5 Simple User-Defined Functions.- 3.6 Applications.- 3.7 Debugging Your Programs.- 3.8 Exercises.- 4 Selection and Repetition Constructs.- 4.1 Relational and Logical Operators.- 4.2 Selection (IF...THEN...ELSE...) Constructs.- 4.3 Choosing Alternatives From a List of Possibilities.- 4.4 Repetition (LOOP...) Constructs.- 4.5 Applications.- 4.6 Debugging Your Programs.- 4.7 Exercises.- 5 More About Modular Programming.- 5.1 Defining Information Interfaces in C.- 5.2 Menu-Driven Programs.- 5.3 More About Function Interfaces.- 5.4 Recursive Functions.- 5.5 Using Prewritten Code Modules.- 5.6 Using Functions as Arguments and Parameters.- 5.7 Passing Arguments to the main Function.- 5.8 Applications.- 5.9 Debugging Your Programs.- 5.10 Exercises.- 6 Arrays.- 6.1 Arrays in Structured Programming.- 6.2 One-Dimensional Array Implementation in C.- 6.3 Using Arrays in Function Calls.- 6.4 Multidimensional Arrays.- 6.5 Accessing Arrays With Pointers.- 6.6 More About Strings.- 6.8 Debugging Your Programs.- 6.9 Exercises.- 7 User-Defined Data Objects.- 7.1 Creating User-Defined Data Objects.- 7.2 Arrays of Structures.- 7.3 Functions With Structures as Parametersand Data Types.- 7.4 Applications.- 7.5 Debugging Your Programs.- 7.6 Exercises.- 8 Searching and Sorting Algorithms.- 8.1 Introduction.- 8.2 Searching Algorithms.- 8.3 Sorting Algorithms.- 8.5 Application: Merging Sorted Lists.- 8.6 Debugging Your Programs.- 8.7 Exercises.- 9 Basic Statistics and Numerical Analysis.- 9.1 Introduction.- 9.2 Basic Descriptive Statistics.- 9.3 Numerical Differentiation.- 9.4 Numerical Integration.- 9.5 Solving Systems of Linear Equations.- 9.6 Finding the Roots of Equations.- 9.7 Numerical Solutions to Differential Equations.- 9.8 Exercises.- 10 Binary Files, Random Access, and Dynamic Allocation.- 10.1 Binary and Random Access Files.- 10.2 Dynamic Allocation and Linked Lists.- 10.3 Queues and Stacks.- 10.4 Application: Managing Data From Remote Instruments.- 10.5 Exercises.- Appendices.- Appendix 1: Table of ASCII Characters for Windows/DOS-Based PCs.- Appendix 2: Program Listings by Chapter.- Appendix 3: Glossary.
1 Programming Preliminaries.- 1.1 A Five-Step Problem-Solving Process.- 1.2 Defining a Pseudocode Language for Algorithm Development.- 1.3 Organizing Pseudocode Into a Program.- 1.4 Examples.- 1.5 What Is the Point of Programming?.- 1.6 Your First C Program.- 2 The Basics of C Programming.- 2.1 C Program Layout.- 2.2 Basic Input and Output.- 2.3 Reading External Text Files of Unknown Length.- 2.4 Reading a File One Character at a Time.- 2.5 Applications.- 2.6 Debugging Your Programs.- 3 Data Types, Operators, and Functions.- 3.1 Specifying and Using Data Types.- 3.2 Operators.- 3.3 Type Casting.- 3.4 Intrinsic Functions.- 3.5 Simple User-Defined Functions.- 3.6 Applications.- 3.7 Debugging Your Programs.- 3.8 Exercises.- 4 Selection and Repetition Constructs.- 4.1 Relational and Logical Operators.- 4.2 Selection (IF...THEN...ELSE...) Constructs.- 4.3 Choosing Alternatives From a List of Possibilities.- 4.4 Repetition (LOOP...) Constructs.- 4.5 Applications.- 4.6 Debugging Your Programs.- 4.7 Exercises.- 5 More About Modular Programming.- 5.1 Defining Information Interfaces in C.- 5.2 Menu-Driven Programs.- 5.3 More About Function Interfaces.- 5.4 Recursive Functions.- 5.5 Using Prewritten Code Modules.- 5.6 Using Functions as Arguments and Parameters.- 5.7 Passing Arguments to the main Function.- 5.8 Applications.- 5.9 Debugging Your Programs.- 5.10 Exercises.- 6 Arrays.- 6.1 Arrays in Structured Programming.- 6.2 One-Dimensional Array Implementation in C.- 6.3 Using Arrays in Function Calls.- 6.4 Multidimensional Arrays.- 6.5 Accessing Arrays With Pointers.- 6.6 More About Strings.- 6.8 Debugging Your Programs.- 6.9 Exercises.- 7 User-Defined Data Objects.- 7.1 Creating User-Defined Data Objects.- 7.2 Arrays of Structures.- 7.3 Functions With Structures as Parametersand Data Types.- 7.4 Applications.- 7.5 Debugging Your Programs.- 7.6 Exercises.- 8 Searching and Sorting Algorithms.- 8.1 Introduction.- 8.2 Searching Algorithms.- 8.3 Sorting Algorithms.- 8.5 Application: Merging Sorted Lists.- 8.6 Debugging Your Programs.- 8.7 Exercises.- 9 Basic Statistics and Numerical Analysis.- 9.1 Introduction.- 9.2 Basic Descriptive Statistics.- 9.3 Numerical Differentiation.- 9.4 Numerical Integration.- 9.5 Solving Systems of Linear Equations.- 9.6 Finding the Roots of Equations.- 9.7 Numerical Solutions to Differential Equations.- 9.8 Exercises.- 10 Binary Files, Random Access, and Dynamic Allocation.- 10.1 Binary and Random Access Files.- 10.2 Dynamic Allocation and Linked Lists.- 10.3 Queues and Stacks.- 10.4 Application: Managing Data From Remote Instruments.- 10.5 Exercises.- Appendices.- Appendix 1: Table of ASCII Characters for Windows/DOS-Based PCs.- Appendix 2: Program Listings by Chapter.- Appendix 3: Glossary.