35,95 €
35,95 €
inkl. MwSt.
Sofort per Download lieferbar
18 °P sammeln
35,95 €
Als Download kaufen
35,95 €
inkl. MwSt.
Sofort per Download lieferbar
18 °P sammeln
Jetzt verschenken
Alle Infos zum eBook verschenken
35,95 €
inkl. MwSt.
Sofort per Download lieferbar
Alle Infos zum eBook verschenken
18 °P sammeln
- Format: PDF
- Merkliste
- Auf die Merkliste
- Bewerten Bewerten
- Teilen
- Produkt teilen
- Produkterinnerung
- Produkterinnerung
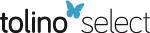
Bitte loggen Sie sich zunächst in Ihr Kundenkonto ein oder registrieren Sie sich bei
bücher.de, um das eBook-Abo tolino select nutzen zu können.
Hier können Sie sich einloggen
Hier können Sie sich einloggen
Sie sind bereits eingeloggt. Klicken Sie auf 2. tolino select Abo, um fortzufahren.
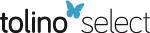
Bitte loggen Sie sich zunächst in Ihr Kundenkonto ein oder registrieren Sie sich bei bücher.de, um das eBook-Abo tolino select nutzen zu können.
Go Recipes: A Problem-Solution Approach provides an invaluable reference to using the Go programming language, showing idiomatic and best practice code for solving common problems encountered by developers in all industries. What you'll learn . Core fundamentals of Go programming language. . How to persist data into relational databases and into NoSQL databases. . How to build scalable backend APIs in Go. . How to build and deploy Go web applications into multiple Cloud platforms. . Building Microservices in the container era.
- Geräte: PC
- ohne Kopierschutz
- eBook Hilfe
- Größe: 6.41MB
Andere Kunden interessierten sich auch für
- Malay MandalRuby Recipes (eBook, PDF)20,95 €
- Shirish ChavanC Recipes (eBook, PDF)43,95 €
- Shiju VargheseWeb Development with Go (eBook, PDF)43,95 €
- Manish SharmaCosmos DB for MongoDB Developers (eBook, PDF)26,95 €
- Deepak VohraPro Docker (eBook, PDF)35,95 €
- Cory GackenheimerNode.js Recipes (eBook, PDF)27,95 €
- Zhimin ZhanSelenium WebDriver Recipes in C# (eBook, PDF)43,95 €
-
-
-
Go Recipes: A Problem-Solution Approach provides an invaluable reference to using the Go programming language, showing idiomatic and best practice code for solving common problems encountered by developers in all industries.
What you'll learn
. Core fundamentals of Go programming language.
. How to persist data into relational databases and into NoSQL databases.
. How to build scalable backend APIs in Go.
. How to build and deploy Go web applications into multiple Cloud platforms.
. Building Microservices in the container era.
Dieser Download kann aus rechtlichen Gründen nur mit Rechnungsadresse in A, B, BG, CY, CZ, D, DK, EW, E, FIN, F, GR, HR, H, IRL, I, LT, L, LR, M, NL, PL, P, R, S, SLO, SK ausgeliefert werden.
Produktdetails
- Produktdetails
- Verlag: Apress
- Seitenzahl: 237
- Erscheinungstermin: 17. November 2016
- Englisch
- ISBN-13: 9781484211885
- Artikelnr.: 53153037
- Verlag: Apress
- Seitenzahl: 237
- Erscheinungstermin: 17. November 2016
- Englisch
- ISBN-13: 9781484211885
- Artikelnr.: 53153037
- Herstellerkennzeichnung Die Herstellerinformationen sind derzeit nicht verfügbar.
Shiju Varghese is a solutions architect who specializes in cloud computing solutions and distributed web apps. His current technology focus is on Go, Google Cloud, Azure, and Docker. He has been working with Web technologies since early 2000, and has developed web apps and distributed systems in Go, C#, and Node.js. His areas of interest include cloud solutions architecture, application containers, distributed systems, RESTful microservice architecture, and mobile backend as a service. Shiju has been awarded Microsoft MVP status five times. He is a regular speaker at various technology conferences.
1. Go Idioms
2. Working with Text
3. Dates and Times
4. Concurrency and Locking
5. Files and IO
6. Graphics and Images
7. Building Cloud Services
8. Writing Web Services
9. Algorithms and Data Structures
10. Memory Management
11. Numeric Computing
12. Big Data Analytics
13. Mobile
14. Windows
15. Testing and Debugging
16. Performance
17. Security and Encryption
18. Databases
19. Parsing Go Programs
20. Gotchas
1. Go Idioms
Common programming patterns in Go. Naming and packaging code. Error handling.
2. Working with Text
Manipulating and formatting strings. Character sets and encodings. Templating text.
3. Dates and Times
Parsing and formatting dates and times. Using timers to schedule events. Running code at precise intervals.
4. Concurrency and Locking
Using goroutines to create multithreaded programs. Use channels to coordinate and synchronise threads. Using locks and atomic operations to safely share data between threads.
5. Files and IO
Reading, writing and processing files. Walking file systems and searching for files. Parsing CSV, JSON and XML.
6. Graphics and Images
Reading images and then resizing, compositing and filtering them. Drawing shapes and text. Converting image formats.
7. Building Cloud Services
Writing cloud services with TCP and UDP. Downloading, uploading and processing files, sending email, implementing network protocols.
8. Writing Web Services
Creating a web server with request routing. Handling cookies and serving multiple file types. Writing server logs and recording performance metrics. Using JSON and WebSockets to interact with Javascript browser applications.
9. Algorithms and Data Structures
Sorting and searching data. Writing trees, graphs and lists to organise data.
10. Memory Management
Understanding and avoiding garbage collection. Efficient use of memory. Working with large datasets.
11. Numeric Computing
Writing statistical functions, working with matrices. Using high precision and complex numbers.
12. Big Data Analytics
Performing calculations over streams of data and time series. Map/reduce and dealing with data larger than memory. Parallelizing data analysis.
13. Mobile
How to write programs and games for mobile devices. Compiling Go programs for Raspberry Pi and other devices.
14. Windows
Creating GUIs on Windows and other operating systems. Writing Windows specific code and calling DLLs.
15. Testing and Debugging
Writing tests and test suites. Separating and running different types of test. Using a debugger with Go programs. Using environment variables for runtime debugging.
16. Performance
Benchmarking Go code. Analysing memory use and performance. Optimisation techniques for Go programs. Tuning concurrency options.
17. Security and Encryption
Hashing and verifying passwords with bcrypt. Computing hashes of data streams. Encrypting and decrypting data. Accessing secure cloud services.
18. Databases
Accessing and querying databases such as MongoDB, mySQL, Postgesql, MS SQL Server and Oracle. Using in-memory databases.
19. Parsing Go Programs
Analysing, formatting and checking Go source code. Generating and templating code.
20. Gotchas
Common pitfalls and subtleties of programming with Go.
2. Working with Text
3. Dates and Times
4. Concurrency and Locking
5. Files and IO
6. Graphics and Images
7. Building Cloud Services
8. Writing Web Services
9. Algorithms and Data Structures
10. Memory Management
11. Numeric Computing
12. Big Data Analytics
13. Mobile
14. Windows
15. Testing and Debugging
16. Performance
17. Security and Encryption
18. Databases
19. Parsing Go Programs
20. Gotchas
1. Go Idioms
Common programming patterns in Go. Naming and packaging code. Error handling.
2. Working with Text
Manipulating and formatting strings. Character sets and encodings. Templating text.
3. Dates and Times
Parsing and formatting dates and times. Using timers to schedule events. Running code at precise intervals.
4. Concurrency and Locking
Using goroutines to create multithreaded programs. Use channels to coordinate and synchronise threads. Using locks and atomic operations to safely share data between threads.
5. Files and IO
Reading, writing and processing files. Walking file systems and searching for files. Parsing CSV, JSON and XML.
6. Graphics and Images
Reading images and then resizing, compositing and filtering them. Drawing shapes and text. Converting image formats.
7. Building Cloud Services
Writing cloud services with TCP and UDP. Downloading, uploading and processing files, sending email, implementing network protocols.
8. Writing Web Services
Creating a web server with request routing. Handling cookies and serving multiple file types. Writing server logs and recording performance metrics. Using JSON and WebSockets to interact with Javascript browser applications.
9. Algorithms and Data Structures
Sorting and searching data. Writing trees, graphs and lists to organise data.
10. Memory Management
Understanding and avoiding garbage collection. Efficient use of memory. Working with large datasets.
11. Numeric Computing
Writing statistical functions, working with matrices. Using high precision and complex numbers.
12. Big Data Analytics
Performing calculations over streams of data and time series. Map/reduce and dealing with data larger than memory. Parallelizing data analysis.
13. Mobile
How to write programs and games for mobile devices. Compiling Go programs for Raspberry Pi and other devices.
14. Windows
Creating GUIs on Windows and other operating systems. Writing Windows specific code and calling DLLs.
15. Testing and Debugging
Writing tests and test suites. Separating and running different types of test. Using a debugger with Go programs. Using environment variables for runtime debugging.
16. Performance
Benchmarking Go code. Analysing memory use and performance. Optimisation techniques for Go programs. Tuning concurrency options.
17. Security and Encryption
Hashing and verifying passwords with bcrypt. Computing hashes of data streams. Encrypting and decrypting data. Accessing secure cloud services.
18. Databases
Accessing and querying databases such as MongoDB, mySQL, Postgesql, MS SQL Server and Oracle. Using in-memory databases.
19. Parsing Go Programs
Analysing, formatting and checking Go source code. Generating and templating code.
20. Gotchas
Common pitfalls and subtleties of programming with Go.
1. Go Idioms
2. Working with Text
3. Dates and Times
4. Concurrency and Locking
5. Files and IO
6. Graphics and Images
7. Building Cloud Services
8. Writing Web Services
9. Algorithms and Data Structures
10. Memory Management
11. Numeric Computing
12. Big Data Analytics
13. Mobile
14. Windows
15. Testing and Debugging
16. Performance
17. Security and Encryption
18. Databases
19. Parsing Go Programs
20. Gotchas
1. Go Idioms
Common programming patterns in Go. Naming and packaging code. Error handling.
2. Working with Text
Manipulating and formatting strings. Character sets and encodings. Templating text.
3. Dates and Times
Parsing and formatting dates and times. Using timers to schedule events. Running code at precise intervals.
4. Concurrency and Locking
Using goroutines to create multithreaded programs. Use channels to coordinate and synchronise threads. Using locks and atomic operations to safely share data between threads.
5. Files and IO
Reading, writing and processing files. Walking file systems and searching for files. Parsing CSV, JSON and XML.
6. Graphics and Images
Reading images and then resizing, compositing and filtering them. Drawing shapes and text. Converting image formats.
7. Building Cloud Services
Writing cloud services with TCP and UDP. Downloading, uploading and processing files, sending email, implementing network protocols.
8. Writing Web Services
Creating a web server with request routing. Handling cookies and serving multiple file types. Writing server logs and recording performance metrics. Using JSON and WebSockets to interact with Javascript browser applications.
9. Algorithms and Data Structures
Sorting and searching data. Writing trees, graphs and lists to organise data.
10. Memory Management
Understanding and avoiding garbage collection. Efficient use of memory. Working with large datasets.
11. Numeric Computing
Writing statistical functions, working with matrices. Using high precision and complex numbers.
12. Big Data Analytics
Performing calculations over streams of data and time series. Map/reduce and dealing with data larger than memory. Parallelizing data analysis.
13. Mobile
How to write programs and games for mobile devices. Compiling Go programs for Raspberry Pi and other devices.
14. Windows
Creating GUIs on Windows and other operating systems. Writing Windows specific code and calling DLLs.
15. Testing and Debugging
Writing tests and test suites. Separating and running different types of test. Using a debugger with Go programs. Using environment variables for runtime debugging.
16. Performance
Benchmarking Go code. Analysing memory use and performance. Optimisation techniques for Go programs. Tuning concurrency options.
17. Security and Encryption
Hashing and verifying passwords with bcrypt. Computing hashes of data streams. Encrypting and decrypting data. Accessing secure cloud services.
18. Databases
Accessing and querying databases such as MongoDB, mySQL, Postgesql, MS SQL Server and Oracle. Using in-memory databases.
19. Parsing Go Programs
Analysing, formatting and checking Go source code. Generating and templating code.
20. Gotchas
Common pitfalls and subtleties of programming with Go.
2. Working with Text
3. Dates and Times
4. Concurrency and Locking
5. Files and IO
6. Graphics and Images
7. Building Cloud Services
8. Writing Web Services
9. Algorithms and Data Structures
10. Memory Management
11. Numeric Computing
12. Big Data Analytics
13. Mobile
14. Windows
15. Testing and Debugging
16. Performance
17. Security and Encryption
18. Databases
19. Parsing Go Programs
20. Gotchas
1. Go Idioms
Common programming patterns in Go. Naming and packaging code. Error handling.
2. Working with Text
Manipulating and formatting strings. Character sets and encodings. Templating text.
3. Dates and Times
Parsing and formatting dates and times. Using timers to schedule events. Running code at precise intervals.
4. Concurrency and Locking
Using goroutines to create multithreaded programs. Use channels to coordinate and synchronise threads. Using locks and atomic operations to safely share data between threads.
5. Files and IO
Reading, writing and processing files. Walking file systems and searching for files. Parsing CSV, JSON and XML.
6. Graphics and Images
Reading images and then resizing, compositing and filtering them. Drawing shapes and text. Converting image formats.
7. Building Cloud Services
Writing cloud services with TCP and UDP. Downloading, uploading and processing files, sending email, implementing network protocols.
8. Writing Web Services
Creating a web server with request routing. Handling cookies and serving multiple file types. Writing server logs and recording performance metrics. Using JSON and WebSockets to interact with Javascript browser applications.
9. Algorithms and Data Structures
Sorting and searching data. Writing trees, graphs and lists to organise data.
10. Memory Management
Understanding and avoiding garbage collection. Efficient use of memory. Working with large datasets.
11. Numeric Computing
Writing statistical functions, working with matrices. Using high precision and complex numbers.
12. Big Data Analytics
Performing calculations over streams of data and time series. Map/reduce and dealing with data larger than memory. Parallelizing data analysis.
13. Mobile
How to write programs and games for mobile devices. Compiling Go programs for Raspberry Pi and other devices.
14. Windows
Creating GUIs on Windows and other operating systems. Writing Windows specific code and calling DLLs.
15. Testing and Debugging
Writing tests and test suites. Separating and running different types of test. Using a debugger with Go programs. Using environment variables for runtime debugging.
16. Performance
Benchmarking Go code. Analysing memory use and performance. Optimisation techniques for Go programs. Tuning concurrency options.
17. Security and Encryption
Hashing and verifying passwords with bcrypt. Computing hashes of data streams. Encrypting and decrypting data. Accessing secure cloud services.
18. Databases
Accessing and querying databases such as MongoDB, mySQL, Postgesql, MS SQL Server and Oracle. Using in-memory databases.
19. Parsing Go Programs
Analysing, formatting and checking Go source code. Generating and templating code.
20. Gotchas
Common pitfalls and subtleties of programming with Go.