Alle Infos zum eBook verschenken
- Format: ePub
- Merkliste
- Auf die Merkliste
- Bewerten Bewerten
- Teilen
- Produkt teilen
- Produkterinnerung
- Produkterinnerung
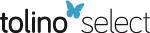
Hier können Sie sich einloggen
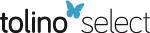
Bitte loggen Sie sich zunächst in Ihr Kundenkonto ein oder registrieren Sie sich bei bücher.de, um das eBook-Abo tolino select nutzen zu können.
There are lots of introductory C books, but this is the first one that has the no-nonsense, practical approach that has made Nutshell Handbooks famous.C programming is more than just getting the syntax right. Style and debugging also play a tremendous part in creating programs that run well and are easy to maintain. This book teaches you not only the mechanics of programming, but also describes how to create programs that are easy to read, debug, and update.Practical rules are stressed. For example, there are fifteen precedence rules in C (&& comes before comes before ?:). The practical…mehr
- Geräte: eReader
- mit Kopierschutz
- eBook Hilfe
- Größe: 3.88MB
- Steve OuallinePractical C++ Programming (eBook, ePUB)26,95 €
- Robert C. SeacordEffective C (eBook, ePUB)24,95 €
- Stephen PrataC Primer Plus (eBook, ePUB)20,95 €
- Damian ConwayPerl Best Practices (eBook, ePUB)19,95 €
- David GriffithsHead First C (eBook, ePUB)31,95 €
- John CalcoteAutotools, 2nd Edition (eBook, ePUB)24,95 €
- Bradley JonesC Programming in One Hour a Day, Sams Teach Yourself (eBook, ePUB)13,95 €
-
-
-
Dieser Download kann aus rechtlichen Gründen nur mit Rechnungsadresse in A, B, BG, CY, CZ, D, DK, EW, E, FIN, F, GR, HR, H, IRL, I, LT, L, LR, M, NL, PL, P, R, S, SLO, SK ausgeliefert werden.
- Produktdetails
- Verlag: O'Reilly Media
- Seitenzahl: 456
- Erscheinungstermin: 1. August 1997
- Englisch
- ISBN-13: 9781449313043
- Artikelnr.: 40529939
- Verlag: O'Reilly Media
- Seitenzahl: 456
- Erscheinungstermin: 1. August 1997
- Englisch
- ISBN-13: 9781449313043
- Artikelnr.: 40529939
How This Book is Organized
Chapter by Chapter
Notes on the Third Edition
Font Conventions
Obtaining Source Code
Comments and Questions
Acknowledgments
Acknowledgments to the Third Edition
Basics
Chapter 1: What Is C?
1.1 How Programming Works
1.2 Brief History of C
1.3 How C Works
1.4 How to Learn C
Chapter 2: Basics of Program Writing
2.1 Programs from Conception to Execution
2.2 Creating a Real Program
2.3 Creating a Program Using a Command-Line Compiler
2.4 Creating a Program Using an Integrated Development Environment
2.5 Getting Help on UNIX
2.6 Getting Help in an Integrated Development Environment
2.7 IDE Cookbooks
2.8 Programming Exercises
Chapter 3: Style
3.1 Common Coding Practices
3.2 Coding Religion
3.3 Indentation and Code Format
3.4 Clarity
3.5 Simplicity
3.6 Summary
Chapter 4: Basic Declarations and Expressions
4.1 Elements of a Program
4.2 Basic Program Structure
4.3 Simple Expressions
4.4 Variables and Storage
4.5 Variable Declarations
4.6 Integers
4.7 Assignment Statements
4.8 printf Function
4.9 Floating Point
4.10 Floating Point Versus Integer Divide
4.11 Characters
4.12 Answers
4.13 Programming Exercises
Chapter 5: Arrays, Qualifiers, and Reading Numbers
5.1 Arrays
5.2 Strings
5.3 Reading Strings
5.4 Multidimensional Arrays
5.5 Reading Numbers
5.6 Initializing Variables
5.7 Types of Integers
5.8 Types of Floats
5.9 Constant Declarations
5.10 Hexadecimal and Octal Constants
5.11 Operators for Performing Shortcuts
5.12 Side Effects
5.13 ++x or x++
5.14 More Side-Effect Problems
5.15 Answers
5.16 Programming Exercises
Chapter 6: Decision and Control Statements
6.1 if Statement
6.2 else Statement
6.3 How Not to Use strcmp
6.4 Looping Statements
6.5 while Statement
6.6 break Statement
6.7 continue Statement
6.8 Assignment Anywhere Side Effect
6.9 Answer
6.10 Programming Exercises
Chapter 7: Programming Process
7.1 Setting Up
7.2 Specification
7.3 Code Design
7.4 Prototype
7.5 Makefile
7.6 Testing
7.7 Debugging
7.8 Maintenance
7.9 Revisions
7.10 Electronic Archaeology
7.11 Marking Up the Program
7.12 Using the Debugger
7.13 Text Editor as a Browser
7.14 Add Comments
7.15 Programming Exercises
Simple Programming
Chapter 8: More Control Statements
8.1 for Statement
8.2 switch Statement
8.3 switch, break, and continue
8.4 Answers
8.5 Programming Exercises
Chapter 9: Variable Scope and Functions
9.1 Scope and Class
9.2 Functions
9.3 Functions with No Parameters
9.4 Structured Programming
9.5 Recursion
9.6 Answers
9.7 Programming Exercises
Chapter 10: C Preprocessor
10.1 #define Statement
10.2 Conditional Compilation
10.3 include Files
10.4 Parameterized Macros
10.5 Advanced Features
10.6 Summary
10.7 Answers
10.8 Programming Exercises
Chapter 11: Bit Operations
11.1 Bit Operators
11.2 The and Operator (&)
11.3 Bitwise or ( )
11.4 The Bitwise Exclusive or (^)
11.5 The Ones Complement Operator (Not) (~)
11.6 The Left- and Right-Shift Operators ()
11.7 Setting, Clearing, and Testing Bits
11.8 Bitmapped Graphics
11.9 Answers
11.10 Programming Exercises
Chapter 12: Advanced Types
12.1 Structures
12.2 Unions
12.3 typedef
12.4 enum Type
12.5 Casting
12.6 Bit Fields or Packed Structures
12.7 Arrays of Structures
12.8 Summary
12.9 Programming Exercises
Chapter 13: Simple Pointers
13.1 Pointers as Function Arguments
13.2 const Pointers
13.3 Pointers and Arrays
13.4 How Not to Use Pointers
13.5 Using Pointers to Split a String
13.6 Pointers and Structures
13.7 Command-Line Arguments
13.8 Programming Exercises
13.9 Answers
Chapter 14: File Input/Output
14.1 Conversion Routines
14.2 Binary and ASCII Files
14.3 The End-of-Line Puzzle
14.4 Binary I/O
14.5 Buffering Problems
14.6 Unbuffered I/O
14.7 Designing File Formats
14.8 Answers
14.9 Programming Exercises
Chapter 15: Debugging and Optimization
15.1 Debugging
15.2 Interactive Debuggers
15.3 Debugging a Binary Search
15.4 Runtime Errors
15.5 The Confessional Method of Debugging
15.6 Optimization
15.7 Answers
15.8 Programming Exercises
Chapter 16: Floating Point
16.1 Floating-Point Format
16.2 Floating Addition/Subtraction
16.3 Multiplication
16.4 Division
16.5 Overflow and Underflow
16.6 Roundoff Error
16.7 Accuracy
16.8 Minimizing Roundoff Error
16.9 Determining Accuracy
16.10 Precision and Speed
16.11 Power Series
16.12 Programming Exercises
Advanced Programming Concepts
Chapter 17: Advanced Pointers
17.1 Pointers and Structures
17.2 free Function
17.3 Linked List
17.4 Structure Pointer Operator
17.5 Ordered Linked Lists
17.6 Double-Linked Lists
17.7 Trees
17.8 Printing a Tree
17.9 Rest of Program
17.10 Data Structures for a Chess Program
17.11 Answers
17.12 Programming Exercises
Chapter 18: Modular Programming
18.1 Modules
18.2 Public and Private
18.3 The extern Modifier
18.4 Headers
18.5 The Body of the Module
18.6 A Program to Use Infinite Arrays
18.7 The Makefile for Multiple Files
18.8 Using the Infinite Array
18.9 Dividing a Task into Modules
18.10 Module Division Example: Text Editor
18.11 Compiler
18.12 Spreadsheet
18.13 Module Design Guidelines
18.14 Programming Exercises
Chapter 19: Ancient Compilers
19.1 K&R-Style Functions
19.2 Library Changes
19.3 Missing Features
19.4 Free/Malloc Changes
19.5 lint
19.6 Answers
Chapter 20: Portability Problems
20.1 Modularity
20.2 Word Size
20.3 Byte Order Problem
20.4 Alignment Problem
20.5 NULL Pointer Problem
20.6 Filename Problems
20.7 File Types
20.8 Summary
20.9 Answers
Chapter 21: C's Dustier Corners
21.1 do/while
21.2 goto
21.3 The ?: Construct
21.4 The , Operator
21.5 volatile Qualifier
21.6 Answer
Chapter 22: Putting It All Together
22.1 Requirements
22.2 Specification
22.3 Code Design
22.4 Coding
22.5 Functional Description
22.6 Expandability
22.7 Testing
22.8 Revisions
22.9 A Final Warning
22.10 Program Files
22.11 Programming Exercises
Chapter 23: Programming Adages
23.1 General
23.2 Design
23.3 Declarations
23.4 switch Statement
23.5 Preprocessor
23.6 Style
23.7 Compiling
23.8 Final Note
23.9 Answer
Other Language Features
ASCII Table
Ranges and Parameter Passing Conversions
Ranges
Automatic Type Conversions to Use When Passing Parameters
Operator Precedence Rules
Standard Rules
Practical Subset
A Program to Compute a Sine Using a Power Series
The sine.c Program
Glossary
Colophon
How This Book is Organized
Chapter by Chapter
Notes on the Third Edition
Font Conventions
Obtaining Source Code
Comments and Questions
Acknowledgments
Acknowledgments to the Third Edition
Basics
Chapter 1: What Is C?
1.1 How Programming Works
1.2 Brief History of C
1.3 How C Works
1.4 How to Learn C
Chapter 2: Basics of Program Writing
2.1 Programs from Conception to Execution
2.2 Creating a Real Program
2.3 Creating a Program Using a Command-Line Compiler
2.4 Creating a Program Using an Integrated Development Environment
2.5 Getting Help on UNIX
2.6 Getting Help in an Integrated Development Environment
2.7 IDE Cookbooks
2.8 Programming Exercises
Chapter 3: Style
3.1 Common Coding Practices
3.2 Coding Religion
3.3 Indentation and Code Format
3.4 Clarity
3.5 Simplicity
3.6 Summary
Chapter 4: Basic Declarations and Expressions
4.1 Elements of a Program
4.2 Basic Program Structure
4.3 Simple Expressions
4.4 Variables and Storage
4.5 Variable Declarations
4.6 Integers
4.7 Assignment Statements
4.8 printf Function
4.9 Floating Point
4.10 Floating Point Versus Integer Divide
4.11 Characters
4.12 Answers
4.13 Programming Exercises
Chapter 5: Arrays, Qualifiers, and Reading Numbers
5.1 Arrays
5.2 Strings
5.3 Reading Strings
5.4 Multidimensional Arrays
5.5 Reading Numbers
5.6 Initializing Variables
5.7 Types of Integers
5.8 Types of Floats
5.9 Constant Declarations
5.10 Hexadecimal and Octal Constants
5.11 Operators for Performing Shortcuts
5.12 Side Effects
5.13 ++x or x++
5.14 More Side-Effect Problems
5.15 Answers
5.16 Programming Exercises
Chapter 6: Decision and Control Statements
6.1 if Statement
6.2 else Statement
6.3 How Not to Use strcmp
6.4 Looping Statements
6.5 while Statement
6.6 break Statement
6.7 continue Statement
6.8 Assignment Anywhere Side Effect
6.9 Answer
6.10 Programming Exercises
Chapter 7: Programming Process
7.1 Setting Up
7.2 Specification
7.3 Code Design
7.4 Prototype
7.5 Makefile
7.6 Testing
7.7 Debugging
7.8 Maintenance
7.9 Revisions
7.10 Electronic Archaeology
7.11 Marking Up the Program
7.12 Using the Debugger
7.13 Text Editor as a Browser
7.14 Add Comments
7.15 Programming Exercises
Simple Programming
Chapter 8: More Control Statements
8.1 for Statement
8.2 switch Statement
8.3 switch, break, and continue
8.4 Answers
8.5 Programming Exercises
Chapter 9: Variable Scope and Functions
9.1 Scope and Class
9.2 Functions
9.3 Functions with No Parameters
9.4 Structured Programming
9.5 Recursion
9.6 Answers
9.7 Programming Exercises
Chapter 10: C Preprocessor
10.1 #define Statement
10.2 Conditional Compilation
10.3 include Files
10.4 Parameterized Macros
10.5 Advanced Features
10.6 Summary
10.7 Answers
10.8 Programming Exercises
Chapter 11: Bit Operations
11.1 Bit Operators
11.2 The and Operator (&)
11.3 Bitwise or ( )
11.4 The Bitwise Exclusive or (^)
11.5 The Ones Complement Operator (Not) (~)
11.6 The Left- and Right-Shift Operators ()
11.7 Setting, Clearing, and Testing Bits
11.8 Bitmapped Graphics
11.9 Answers
11.10 Programming Exercises
Chapter 12: Advanced Types
12.1 Structures
12.2 Unions
12.3 typedef
12.4 enum Type
12.5 Casting
12.6 Bit Fields or Packed Structures
12.7 Arrays of Structures
12.8 Summary
12.9 Programming Exercises
Chapter 13: Simple Pointers
13.1 Pointers as Function Arguments
13.2 const Pointers
13.3 Pointers and Arrays
13.4 How Not to Use Pointers
13.5 Using Pointers to Split a String
13.6 Pointers and Structures
13.7 Command-Line Arguments
13.8 Programming Exercises
13.9 Answers
Chapter 14: File Input/Output
14.1 Conversion Routines
14.2 Binary and ASCII Files
14.3 The End-of-Line Puzzle
14.4 Binary I/O
14.5 Buffering Problems
14.6 Unbuffered I/O
14.7 Designing File Formats
14.8 Answers
14.9 Programming Exercises
Chapter 15: Debugging and Optimization
15.1 Debugging
15.2 Interactive Debuggers
15.3 Debugging a Binary Search
15.4 Runtime Errors
15.5 The Confessional Method of Debugging
15.6 Optimization
15.7 Answers
15.8 Programming Exercises
Chapter 16: Floating Point
16.1 Floating-Point Format
16.2 Floating Addition/Subtraction
16.3 Multiplication
16.4 Division
16.5 Overflow and Underflow
16.6 Roundoff Error
16.7 Accuracy
16.8 Minimizing Roundoff Error
16.9 Determining Accuracy
16.10 Precision and Speed
16.11 Power Series
16.12 Programming Exercises
Advanced Programming Concepts
Chapter 17: Advanced Pointers
17.1 Pointers and Structures
17.2 free Function
17.3 Linked List
17.4 Structure Pointer Operator
17.5 Ordered Linked Lists
17.6 Double-Linked Lists
17.7 Trees
17.8 Printing a Tree
17.9 Rest of Program
17.10 Data Structures for a Chess Program
17.11 Answers
17.12 Programming Exercises
Chapter 18: Modular Programming
18.1 Modules
18.2 Public and Private
18.3 The extern Modifier
18.4 Headers
18.5 The Body of the Module
18.6 A Program to Use Infinite Arrays
18.7 The Makefile for Multiple Files
18.8 Using the Infinite Array
18.9 Dividing a Task into Modules
18.10 Module Division Example: Text Editor
18.11 Compiler
18.12 Spreadsheet
18.13 Module Design Guidelines
18.14 Programming Exercises
Chapter 19: Ancient Compilers
19.1 K&R-Style Functions
19.2 Library Changes
19.3 Missing Features
19.4 Free/Malloc Changes
19.5 lint
19.6 Answers
Chapter 20: Portability Problems
20.1 Modularity
20.2 Word Size
20.3 Byte Order Problem
20.4 Alignment Problem
20.5 NULL Pointer Problem
20.6 Filename Problems
20.7 File Types
20.8 Summary
20.9 Answers
Chapter 21: C's Dustier Corners
21.1 do/while
21.2 goto
21.3 The ?: Construct
21.4 The , Operator
21.5 volatile Qualifier
21.6 Answer
Chapter 22: Putting It All Together
22.1 Requirements
22.2 Specification
22.3 Code Design
22.4 Coding
22.5 Functional Description
22.6 Expandability
22.7 Testing
22.8 Revisions
22.9 A Final Warning
22.10 Program Files
22.11 Programming Exercises
Chapter 23: Programming Adages
23.1 General
23.2 Design
23.3 Declarations
23.4 switch Statement
23.5 Preprocessor
23.6 Style
23.7 Compiling
23.8 Final Note
23.9 Answer
Other Language Features
ASCII Table
Ranges and Parameter Passing Conversions
Ranges
Automatic Type Conversions to Use When Passing Parameters
Operator Precedence Rules
Standard Rules
Practical Subset
A Program to Compute a Sine Using a Power Series
The sine.c Program
Glossary
Colophon