Programming Entity Framework (eBook, ePUB)
Building Data Centric Apps with the ADO.NET Entity Framework
Alle Infos zum eBook verschenken
Programming Entity Framework (eBook, ePUB)
Building Data Centric Apps with the ADO.NET Entity Framework
- Format: ePub
- Merkliste
- Auf die Merkliste
- Bewerten Bewerten
- Teilen
- Produkt teilen
- Produkterinnerung
- Produkterinnerung
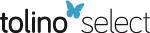
Hier können Sie sich einloggen
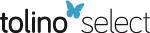
Bitte loggen Sie sich zunächst in Ihr Kundenkonto ein oder registrieren Sie sich bei bücher.de, um das eBook-Abo tolino select nutzen zu können.
Get a thorough introduction to ADO.NET Entity Framework 4 -- Microsoft's core framework for modeling and interacting with data in .NET applications. The second edition of this acclaimed guide provides a hands-on tour of the framework latest version in Visual Studio 2010 and .NET Framework 4. Not only will you learn how to use EF4 in a variety of applications, you'll also gain a deep understanding of its architecture and APIs.Written by Julia Lerman, the leading independent authority on the framework, Programming Entity Framework covers it all -- from the Entity Data Model and Object Services…mehr
- Geräte: eReader
- mit Kopierschutz
- eBook Hilfe
- Größe: 6.98MB
- FamilySharing(5)
- Marco De SanctisEntity Framework 4 in Action (eBook, ePUB)38,56 €
- Julia LermanProgramming Entity Framework: DbContext (eBook, ePUB)11,95 €
- Jignesh TrivediBuilding a Web App with Blazor and ASP .Net Core: Create a Single Page App with Blazor Server and Entity Framework Core (eBook, ePUB)13,99 €
- Julia LermanProgramming Entity Framework: Code First (eBook, ePUB)9,95 €
- Jürgen KotzVisual C# 2019 - Grundlagen, Profiwissen und Rezepte (eBook, ePUB)49,99 €
- Jürgen KotzC# und .NET 6 - Grundlagen, Profiwissen und Rezepte (eBook, ePUB)49,99 €
- Jürgen KotzC# und .NET 8 - Grundlagen, Profiwissen und Rezepte (eBook, ePUB)49,99 €
-
-
-
Dieser Download kann aus rechtlichen Gründen nur mit Rechnungsadresse in A, B, BG, CY, CZ, D, DK, EW, E, FIN, F, GR, HR, H, IRL, I, LT, L, LR, M, NL, PL, P, R, S, SLO, SK ausgeliefert werden.
- Produktdetails
- Verlag: O'Reilly Media
- Seitenzahl: 920
- Erscheinungstermin: 9. August 2010
- Englisch
- ISBN-13: 9781449399658
- Artikelnr.: 37904049
- Verlag: O'Reilly Media
- Seitenzahl: 920
- Erscheinungstermin: 9. August 2010
- Englisch
- ISBN-13: 9781449399658
- Artikelnr.: 37904049
- Herstellerkennzeichnung Die Herstellerinformationen sind derzeit nicht verfügbar.
Preface
Who This Book Is For
How This Book Is Organized
What You Need to Use This Book
This Book's Website
Conventions Used in This Book
Using Code Examples
Safari® Books Online
Comments and Questions
Acknowledgments
Author Note for Third Printing, August 2011
Entity Framework 4.1 (Code First and DbContext) Has Released
Entity Framework June 2011 CTP
Chapter 1: Introducing the ADO.NET Entity Framework
1.1 The Entity Relationship Model: Programming Against a Model, Not the Database
1.2 The Entity Data Model: A Client-Side Data Model
1.3 Entities: Blueprints for Business Classes
1.4 The Backend Database: Your Choice
1.5 Entity Framework Features: APIs and Tools
1.6 The Entity Framework and WCF Services
1.7 What About ADO.NET DataSets and LINQ to SQL?
1.8 Entity Framework Pain Points Are Fading Away
1.9 Programming the Entity Framework
Chapter 2: Exploring the Entity Data Model
2.1 Why Use an Entity Data Model?
2.2 The EDM Within the Entity Framework
2.3 Walkthrough: Building Your First EDM
2.4 Inspecting the EDM in the Designer Window
2.5 The Model's Supporting Metadata
2.6 Viewing the Model in the Model Browser
2.7 Viewing the Model's Raw XML
2.8 CSDL: The Conceptual Schema
2.9 SSDL: The Store Schema
2.10 MSL: The Mappings
2.11 Database Views in the EDM
2.12 Summary
Chapter 3: Querying Entity Data Models
3.1 Query the Model, Not the Database
3.2 Your First EDM Query
3.3 Querying with LINQ to Entities
3.4 Querying with Object Services and Entity SQL
3.5 Querying with Methods
3.6 The Shortest Query
3.7 ObjectQuery, ObjectSet, and LINQ to Entities
3.8 Querying with EntityClient to Return Streamed Data
3.9 Translating Entity Queries to Database Queries
3.10 Avoiding Inadvertent Query Execution
3.11 Summary
Chapter 4: Exploring LINQ to Entities in Greater Depth
4.1 Getting Ready with Some New Lingo
4.2 Projections in Queries
4.3 Projections in LINQ to Entities
4.4 Using Navigations in Queries
4.5 Joins and Nested Queries
4.6 Grouping
4.7 Shaping Data Returned by Queries
4.8 Loading Related Data
4.9 Retrieving a Single Entity
4.10 Finding More Query Samples
4.11 Summary
Chapter 5: Exploring Entity SQL in Greater Depth
5.1 Literals in Entity SQL
5.2 Projecting in Entity SQL
5.3 Using Navigation in Entity SQL Queries
5.4 Using Joins
5.5 Nesting Queries
5.6 Grouping in Entity SQL
5.7 Shaping Data with Entity SQL
5.8 Understanding Entity SQL's Wrapped and Unwrapped Results
5.9 Summary
Chapter 6: Modifying Entities and Saving Changes
6.1 Keeping Track of Entities
6.2 Saving Changes Back to the Database
6.3 Inserting New Objects
6.4 Inserting New Parents and Children
6.5 Deleting Entities
6.6 Summary
Chapter 7: Using Stored Procedures with the EDM
7.1 Updating the Model from a Database
7.2 Working with Functions
7.3 Mapping Functions to Entities
7.4 Using the EDM Designer Model Browser to Import Additional Functions into Your Model
7.5 Mapping the First of the Read Stored Procedures: ContactsbyState
7.6 Mapping a Function to a Scalar Type
7.7 Mapping a Function to a Complex Type
7.8 Summary
Chapter 8: Implementing a More Real-World Model
8.1 Introducing the BreakAway Geek Adventures Business Model and Legacy Database
8.2 Creating a Separate Project for an EDM
8.3 Inspecting and Cleaning Up a New EDM
8.4 Setting Default Values
8.5 Mapping Stored Procedures
8.6 Working with Many-to-Many Relationships
8.7 Inspecting the Completed BreakAway Model
8.8 Building the BreakAway Model Assembly
8.9 Summary
Chapter 9: Data Binding with Windows Forms and WPF Applications
9.1 Data Binding with Windows Forms Applications
9.2 Data Binding with WPF Applications
9.3 Summary
Chapter 10: Working with Object Services
10.1 Where Does Object Services Fit into the Framework?
10.2 Processing Queries
10.3 Materializing Objects
10.4 Managing Object State
10.5 Managing Relationships
10.6 Taking Control of ObjectState
10.7 Sending Changes Back to the Database
10.8 Implementing Serialization, Data Binding, and More
10.9 Summary
Chapter 11: Customizing Entities
11.1 Partial Classes
11.2 Using Partial Methods
11.3 Subscribing to Event Handlers
11.4 Creating Your Own Partial Methods and Properties
11.5 Overriding Default Code Generation
11.6 Summary
Chapter 12: Data Binding with RAD ASP.NET Applications
12.1 Using the EntityDataSource Control to Access Flat Data
12.2 Understanding How the EntityDataSource Retrieves and Updates Your Data
12.3 Working with Related EntityReference Data
12.4 Working with Hierarchical Data in a Master/Detail Form
12.5 Exploring EntityDataSource Events
12.6 Building Dynamic Data Websites
12.7 Summary
Chapter 13: Creating and Using POCO Entities
13.1 Creating POCO Classes
13.2 Change Tracking with POCOs
13.3 Loading Related Data with POCOs
13.4 Exploring and Correcting POCOs' Impact on Two-Way Relationships
13.5 Using Proxies to Enable Change Notification, Lazy Loading, and Relationship Fix-Up
13.6 Using T4 to Generate POCO Classes
13.7 Creating a Model That Works with Preexisting Classes
13.8 Code First: Using Entity Framework with No Model at All
13.9 Summary
Chapter 14: Customizing Entity Data Models Using the EDM Designer
14.1 Mapping Table per Type Inheritance for Tables That Describe Derived Types
14.2 Mapping Unique Foreign Keys
14.3 Mapping an Entity to More Than One Table
14.4 Splitting a Single Table into Multiple Entities
14.5 Filtering Entities with Conditional Mapping
14.6 Implementing Table per Hierarchy Inheritance for Tables That Contain Multiple Types
14.7 Creating Complex Types to Encapsulate Sets of Properties
14.8 Using Additional Customization Options
14.9 Summary
Chapter 15: Defining EDM Mappings That Are Not Supported by the Designer
15.1 Using Model-Defined Functions
15.2 Mapping Table per Concrete (TPC) Type Inheritance for Tables with Overlapping Fields
15.3 Using QueryView to Create Read-Only Entities and Other Specialized Mappings
15.4 Summary
Chapter 16: Gaining Additional Stored Procedure and View Support in the Raw XML
16.1 Reviewing Procedures, Views, and UDFs in the EDM
16.2 Working with Stored Procedures That Return Data
16.3 Executing Queries on Demand with ExecuteStoreQuery
16.4 Adding Native Queries to the Model
16.5 Adding Native Views to the Model
16.6 Using Commands That Affect the Database
16.7 Mapping Insert/Update/Delete to Types Within an Inheritance Structure
16.8 Implementing and Querying with User-Defined Functions (UDFs)
16.9 Summary
Chapter 17: Using EntityObjects in WCF Services
17.1 Planning for an Entity Framework-Agnostic Client
17.2 Building a Simple WCF Service with EntityObjects
17.3 Implementing the Service Interface
17.4 Building a Simple Console App to Consume an EntityObject Service
17.5 Creating WCF Data Services with Entities
17.6 Understanding How WCF RIA Services Relates to the Entity Framework
17.7 Summary
Chapter 18: Using POCOs and Self-Tracking Entities in WCF Services
18.1 Creating WCF-Friendly POCO Classes
18.2 Building a WCF Service That Uses POCO Classes
18.3 Using the Self-Tracking Entities Template for WCF Services
18.4 Using POCO Entities with WCF Data and RIA Services
18.5 Sorting Out the Many Options for Creating Services
18.6 Summary
Chapter 19: Working with Relationships and Associations
19.1 Deconstructing Relationships in the Entity Data Model
19.2 Understanding the Major Differences Between Foreign Key Associations and Independent Associations
19.3 Deconstructing Relationships Between Instantiated Entities
19.4 Defining Relationships Between Entities
19.5 Learning a Few Last Tricks to Make You a Relationship Pro
19.6 Summary
Chapter 20: Real World Apps: Connections, Transactions, Performance, and More
20.1 Entity Framework and Connections
20.2 Fine-Tuning Transactions
20.3 Understanding Security
20.4 Fine-Tuning Performance
20.5 Exploiting Multithreaded Applications
20.6 Exploiting .NET 4 Parallel Computing
20.7 Summary
Chapter 21: Manipulating Entities with ObjectStateManager and MetadataWorkspace
21.1 Manipulating Entities and Their State with ObjectStateManager
21.2 Using ObjectStateManager to Build an EntityState Visualizer
21.3 Using the MetadataWorkspace
21.4 Building Dynamic Queries and Reading Results
21.5 Creating and Manipulating Entities Dynamically
21.6 Summary
Chapter 22: Handling Exceptions
22.1 Preparing for Exceptions
22.2 Handling EntityConnectionString Exceptions
22.3 Handling Query Compilation Exceptions
22.4 Creating a Common Wrapper to Handle Query Execution Exceptions
22.5 Handling Exceptions Thrown During SaveChanges Command Execution
22.6 Handling Concurrency Exceptions
22.7 Summary
Chapter 23: Planning for Concurrency Problems
23.1 Understanding Database Concurrency Conflicts
23.2 Understanding Optimistic Concurrency Options in the Entity Framework
23.3 Implementing Optimistic Concurrency with the Entity Framework
23.4 Handling OptimisticConcurrencyExceptions
23.5 Handling Concurrency Exceptions at a Lower Level
23.6 Handling Exceptions When Transactions Are Your Own
23.7 Summary
Chapter 24: Building Persistent Ignorant, Testable Applications
24.1 Testing the BreakAway Application Components
24.2 Getting Started with Testing
24.3 Creating Persistent Ignorant Entities
24.4 Building Tests That Do Not Hit the Database
24.5 Using the New Infrastructure in Your Application
24.6 Application Architecture Benefits from Designing Testable Code
24.7 Considering Mocking Frameworks?
24.8 Summary
Chapter 25: Domain-Centric Modeling
25.1 Creating a Model and Database Using Model First
25.2 Using the Feature CTP Code-First Add-On
25.3 Using SQL Server Modeling's "M" Language
25.4 Summary
Chapter 26: Using Entities in Layered Client-Side Applications
26.1 Isolating the ObjectContext
26.2 Separating Entity-Specific Logic from ObjectContext Logic
26.3 Working with POCO Entities
26.4 Summary
Chapter 27: Building Layered Web Applications
27.1 Understanding How ObjectContext Fits into the Web Page Life Cycle
27.2 Building an N-Tier Web Forms Application
27.3 Building an ASP.NET MVC Application
27.4 Editing Entities and Graphs on an MVC Application
27.5 Summary
Entity Framework Assemblies and Namespaces
Unpacking the Entity Framework Files
Exploring the Namespaces
Data-Binding with Complex Types
Using Complex Types with ASP.NET EntityDataSource
Identifying Unexpected Behavior When Binding Complex Types
Additional Details About Entity Data Model Metadata
Seeing EDMX Schema Validation in Action
Additional Conceptual Model Details
Additional SSDL Metadata Details
Additional MSL Metadata Details
Colophon
Preface
Who This Book Is For
How This Book Is Organized
What You Need to Use This Book
This Book's Website
Conventions Used in This Book
Using Code Examples
Safari® Books Online
Comments and Questions
Acknowledgments
Author Note for Third Printing, August 2011
Entity Framework 4.1 (Code First and DbContext) Has Released
Entity Framework June 2011 CTP
Chapter 1: Introducing the ADO.NET Entity Framework
1.1 The Entity Relationship Model: Programming Against a Model, Not the Database
1.2 The Entity Data Model: A Client-Side Data Model
1.3 Entities: Blueprints for Business Classes
1.4 The Backend Database: Your Choice
1.5 Entity Framework Features: APIs and Tools
1.6 The Entity Framework and WCF Services
1.7 What About ADO.NET DataSets and LINQ to SQL?
1.8 Entity Framework Pain Points Are Fading Away
1.9 Programming the Entity Framework
Chapter 2: Exploring the Entity Data Model
2.1 Why Use an Entity Data Model?
2.2 The EDM Within the Entity Framework
2.3 Walkthrough: Building Your First EDM
2.4 Inspecting the EDM in the Designer Window
2.5 The Model's Supporting Metadata
2.6 Viewing the Model in the Model Browser
2.7 Viewing the Model's Raw XML
2.8 CSDL: The Conceptual Schema
2.9 SSDL: The Store Schema
2.10 MSL: The Mappings
2.11 Database Views in the EDM
2.12 Summary
Chapter 3: Querying Entity Data Models
3.1 Query the Model, Not the Database
3.2 Your First EDM Query
3.3 Querying with LINQ to Entities
3.4 Querying with Object Services and Entity SQL
3.5 Querying with Methods
3.6 The Shortest Query
3.7 ObjectQuery, ObjectSet, and LINQ to Entities
3.8 Querying with EntityClient to Return Streamed Data
3.9 Translating Entity Queries to Database Queries
3.10 Avoiding Inadvertent Query Execution
3.11 Summary
Chapter 4: Exploring LINQ to Entities in Greater Depth
4.1 Getting Ready with Some New Lingo
4.2 Projections in Queries
4.3 Projections in LINQ to Entities
4.4 Using Navigations in Queries
4.5 Joins and Nested Queries
4.6 Grouping
4.7 Shaping Data Returned by Queries
4.8 Loading Related Data
4.9 Retrieving a Single Entity
4.10 Finding More Query Samples
4.11 Summary
Chapter 5: Exploring Entity SQL in Greater Depth
5.1 Literals in Entity SQL
5.2 Projecting in Entity SQL
5.3 Using Navigation in Entity SQL Queries
5.4 Using Joins
5.5 Nesting Queries
5.6 Grouping in Entity SQL
5.7 Shaping Data with Entity SQL
5.8 Understanding Entity SQL's Wrapped and Unwrapped Results
5.9 Summary
Chapter 6: Modifying Entities and Saving Changes
6.1 Keeping Track of Entities
6.2 Saving Changes Back to the Database
6.3 Inserting New Objects
6.4 Inserting New Parents and Children
6.5 Deleting Entities
6.6 Summary
Chapter 7: Using Stored Procedures with the EDM
7.1 Updating the Model from a Database
7.2 Working with Functions
7.3 Mapping Functions to Entities
7.4 Using the EDM Designer Model Browser to Import Additional Functions into Your Model
7.5 Mapping the First of the Read Stored Procedures: ContactsbyState
7.6 Mapping a Function to a Scalar Type
7.7 Mapping a Function to a Complex Type
7.8 Summary
Chapter 8: Implementing a More Real-World Model
8.1 Introducing the BreakAway Geek Adventures Business Model and Legacy Database
8.2 Creating a Separate Project for an EDM
8.3 Inspecting and Cleaning Up a New EDM
8.4 Setting Default Values
8.5 Mapping Stored Procedures
8.6 Working with Many-to-Many Relationships
8.7 Inspecting the Completed BreakAway Model
8.8 Building the BreakAway Model Assembly
8.9 Summary
Chapter 9: Data Binding with Windows Forms and WPF Applications
9.1 Data Binding with Windows Forms Applications
9.2 Data Binding with WPF Applications
9.3 Summary
Chapter 10: Working with Object Services
10.1 Where Does Object Services Fit into the Framework?
10.2 Processing Queries
10.3 Materializing Objects
10.4 Managing Object State
10.5 Managing Relationships
10.6 Taking Control of ObjectState
10.7 Sending Changes Back to the Database
10.8 Implementing Serialization, Data Binding, and More
10.9 Summary
Chapter 11: Customizing Entities
11.1 Partial Classes
11.2 Using Partial Methods
11.3 Subscribing to Event Handlers
11.4 Creating Your Own Partial Methods and Properties
11.5 Overriding Default Code Generation
11.6 Summary
Chapter 12: Data Binding with RAD ASP.NET Applications
12.1 Using the EntityDataSource Control to Access Flat Data
12.2 Understanding How the EntityDataSource Retrieves and Updates Your Data
12.3 Working with Related EntityReference Data
12.4 Working with Hierarchical Data in a Master/Detail Form
12.5 Exploring EntityDataSource Events
12.6 Building Dynamic Data Websites
12.7 Summary
Chapter 13: Creating and Using POCO Entities
13.1 Creating POCO Classes
13.2 Change Tracking with POCOs
13.3 Loading Related Data with POCOs
13.4 Exploring and Correcting POCOs' Impact on Two-Way Relationships
13.5 Using Proxies to Enable Change Notification, Lazy Loading, and Relationship Fix-Up
13.6 Using T4 to Generate POCO Classes
13.7 Creating a Model That Works with Preexisting Classes
13.8 Code First: Using Entity Framework with No Model at All
13.9 Summary
Chapter 14: Customizing Entity Data Models Using the EDM Designer
14.1 Mapping Table per Type Inheritance for Tables That Describe Derived Types
14.2 Mapping Unique Foreign Keys
14.3 Mapping an Entity to More Than One Table
14.4 Splitting a Single Table into Multiple Entities
14.5 Filtering Entities with Conditional Mapping
14.6 Implementing Table per Hierarchy Inheritance for Tables That Contain Multiple Types
14.7 Creating Complex Types to Encapsulate Sets of Properties
14.8 Using Additional Customization Options
14.9 Summary
Chapter 15: Defining EDM Mappings That Are Not Supported by the Designer
15.1 Using Model-Defined Functions
15.2 Mapping Table per Concrete (TPC) Type Inheritance for Tables with Overlapping Fields
15.3 Using QueryView to Create Read-Only Entities and Other Specialized Mappings
15.4 Summary
Chapter 16: Gaining Additional Stored Procedure and View Support in the Raw XML
16.1 Reviewing Procedures, Views, and UDFs in the EDM
16.2 Working with Stored Procedures That Return Data
16.3 Executing Queries on Demand with ExecuteStoreQuery
16.4 Adding Native Queries to the Model
16.5 Adding Native Views to the Model
16.6 Using Commands That Affect the Database
16.7 Mapping Insert/Update/Delete to Types Within an Inheritance Structure
16.8 Implementing and Querying with User-Defined Functions (UDFs)
16.9 Summary
Chapter 17: Using EntityObjects in WCF Services
17.1 Planning for an Entity Framework-Agnostic Client
17.2 Building a Simple WCF Service with EntityObjects
17.3 Implementing the Service Interface
17.4 Building a Simple Console App to Consume an EntityObject Service
17.5 Creating WCF Data Services with Entities
17.6 Understanding How WCF RIA Services Relates to the Entity Framework
17.7 Summary
Chapter 18: Using POCOs and Self-Tracking Entities in WCF Services
18.1 Creating WCF-Friendly POCO Classes
18.2 Building a WCF Service That Uses POCO Classes
18.3 Using the Self-Tracking Entities Template for WCF Services
18.4 Using POCO Entities with WCF Data and RIA Services
18.5 Sorting Out the Many Options for Creating Services
18.6 Summary
Chapter 19: Working with Relationships and Associations
19.1 Deconstructing Relationships in the Entity Data Model
19.2 Understanding the Major Differences Between Foreign Key Associations and Independent Associations
19.3 Deconstructing Relationships Between Instantiated Entities
19.4 Defining Relationships Between Entities
19.5 Learning a Few Last Tricks to Make You a Relationship Pro
19.6 Summary
Chapter 20: Real World Apps: Connections, Transactions, Performance, and More
20.1 Entity Framework and Connections
20.2 Fine-Tuning Transactions
20.3 Understanding Security
20.4 Fine-Tuning Performance
20.5 Exploiting Multithreaded Applications
20.6 Exploiting .NET 4 Parallel Computing
20.7 Summary
Chapter 21: Manipulating Entities with ObjectStateManager and MetadataWorkspace
21.1 Manipulating Entities and Their State with ObjectStateManager
21.2 Using ObjectStateManager to Build an EntityState Visualizer
21.3 Using the MetadataWorkspace
21.4 Building Dynamic Queries and Reading Results
21.5 Creating and Manipulating Entities Dynamically
21.6 Summary
Chapter 22: Handling Exceptions
22.1 Preparing for Exceptions
22.2 Handling EntityConnectionString Exceptions
22.3 Handling Query Compilation Exceptions
22.4 Creating a Common Wrapper to Handle Query Execution Exceptions
22.5 Handling Exceptions Thrown During SaveChanges Command Execution
22.6 Handling Concurrency Exceptions
22.7 Summary
Chapter 23: Planning for Concurrency Problems
23.1 Understanding Database Concurrency Conflicts
23.2 Understanding Optimistic Concurrency Options in the Entity Framework
23.3 Implementing Optimistic Concurrency with the Entity Framework
23.4 Handling OptimisticConcurrencyExceptions
23.5 Handling Concurrency Exceptions at a Lower Level
23.6 Handling Exceptions When Transactions Are Your Own
23.7 Summary
Chapter 24: Building Persistent Ignorant, Testable Applications
24.1 Testing the BreakAway Application Components
24.2 Getting Started with Testing
24.3 Creating Persistent Ignorant Entities
24.4 Building Tests That Do Not Hit the Database
24.5 Using the New Infrastructure in Your Application
24.6 Application Architecture Benefits from Designing Testable Code
24.7 Considering Mocking Frameworks?
24.8 Summary
Chapter 25: Domain-Centric Modeling
25.1 Creating a Model and Database Using Model First
25.2 Using the Feature CTP Code-First Add-On
25.3 Using SQL Server Modeling's "M" Language
25.4 Summary
Chapter 26: Using Entities in Layered Client-Side Applications
26.1 Isolating the ObjectContext
26.2 Separating Entity-Specific Logic from ObjectContext Logic
26.3 Working with POCO Entities
26.4 Summary
Chapter 27: Building Layered Web Applications
27.1 Understanding How ObjectContext Fits into the Web Page Life Cycle
27.2 Building an N-Tier Web Forms Application
27.3 Building an ASP.NET MVC Application
27.4 Editing Entities and Graphs on an MVC Application
27.5 Summary
Entity Framework Assemblies and Namespaces
Unpacking the Entity Framework Files
Exploring the Namespaces
Data-Binding with Complex Types
Using Complex Types with ASP.NET EntityDataSource
Identifying Unexpected Behavior When Binding Complex Types
Additional Details About Entity Data Model Metadata
Seeing EDMX Schema Validation in Action
Additional Conceptual Model Details
Additional SSDL Metadata Details
Additional MSL Metadata Details
Colophon